Platform-specific code generation
Description of the platform-specific code generation capabilities of Schematic Editor when using C-code export.
Platform-specific code generation allows C code generation for a custom hardware platform. The platform is registered by adding a platform description file into the appropriate directory.
Platform-specific file format
-
id
(mandatory) - unique ID for the new platform, name
(mandatory) - pretty name for the platform,vendor
(optional) - vendor name,description
(optional) - short description,type_mappings
(mandatory) - dictionary of type mappings, with the following keys:int
(mandatory) - type mapping forint
Typhoon type. Value must be a list.uint
(mandatory) - type mapping foruint
Typhoon type. Value must be a list.real
(mandatory) - type mapping forreal
Typhoon type. Value must be a list.
Below is an example of a minimalistic, valid platform description file:
{
"id": "cst",
"name": "Custom Platform A1",
"type_mappings": {
"int": ["short", "int", "long"],
"real": ["float", "double"],
"uint": ["unsigned int"]
}
}
hil
and
vhil
are reserved and platform definition files with these IDs
will not be loaded.Platform description file location
A platform is registered by adding a platform description file into the appropriate directory. By default, Schematic Editor will collect all files from the following locations:
- Windows:
%appdata%\typhoon\<sw_version>\user-platforms
- Linux:
~/.local/share/typhoon/<sw_version>/user-platforms
A platform description file can be also placed in any location defined in the User libraries path settings dialog (File > Modify library paths), as shown in Figure 1.
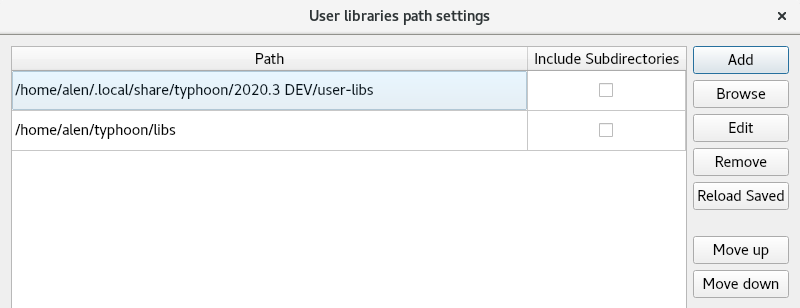
To check if platform definition files are loaded correctly, open Model Settings dialog and go to C code export tab. You'll be able to select your platform in the Platform (beta) combobox (Figure 2 ).
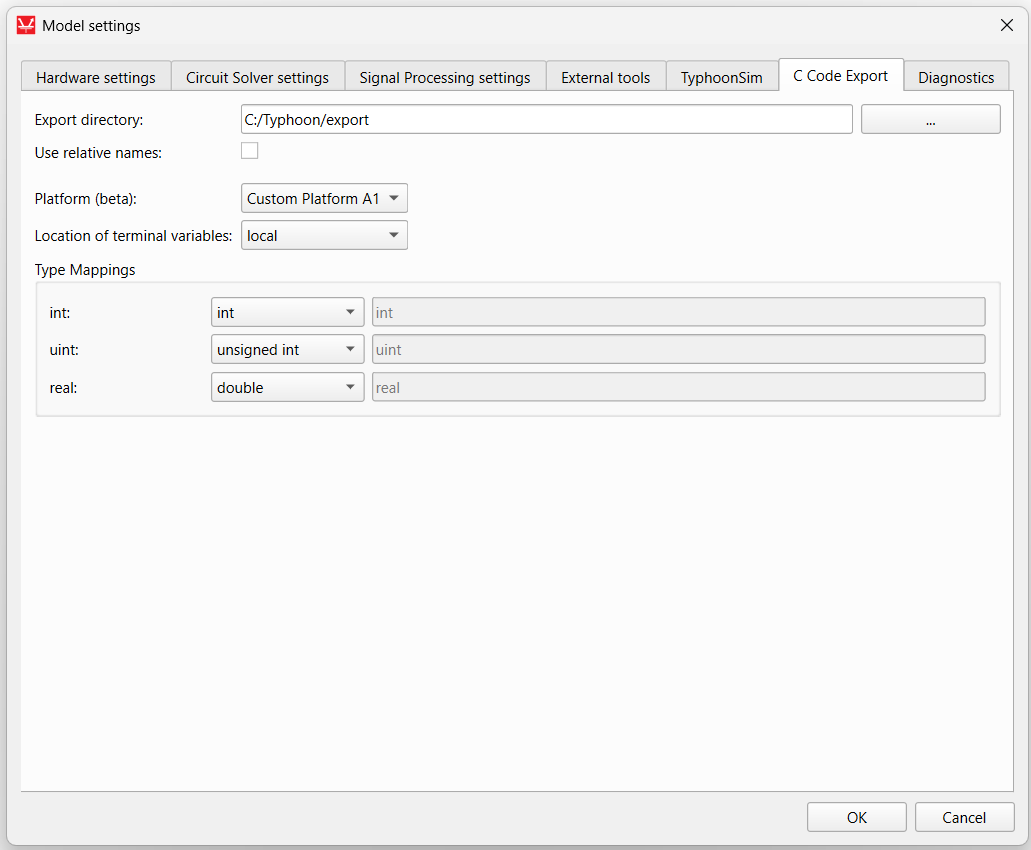
Platform-specific component
A component can be either platform-independent (generic) or platform-dependent. Generic components work on any platform. However, platform-dependent components can work only on a specific platform(s).
To define a platform-dependent component, you need to define the
_supported_platforms
property on the component's mask,
as shown in Figure 3.
In the figure, note that the platforms that are supported are specified by setting the property
default value as a list of platform IDs (in this case, 'cst' for "Custom Platform A1" as
defined in the Platform description file location section.
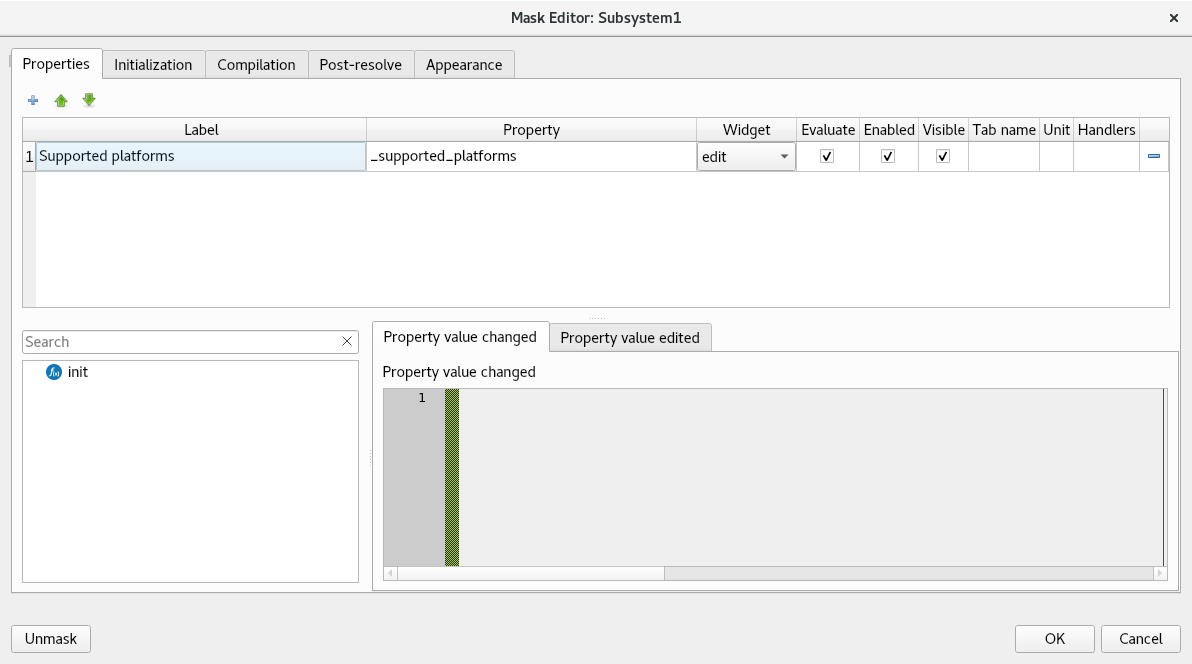
Platform-specific code in handlers
In order to implement platform-specific behavior for a certain component, use the
platform_id
variable that is provided into handlers.
platform_id
is injected in the namespace at
the beginning of compilation/export.For example:
if platform_id == "cst":
mdl.set_property_value(mdl.prop(container_handle, "value"), 1)
else:
mdl.set_property_value(mdl.prop(container_handle, "value"), 2)
Platform-specific C function
platform_id
by defining it as a
parameter of name platform_id
and type str
in the
parameters tab of the general tab inside the C function component. The
platform_id
parameter, containing a string literal, can be used
freely, for example in the output_fnc
as shown
below:// output_fnc // Always use strcmp for string comparisons // Here it is okay to compare using == because platform_id is a C Function parameter // and it will be replaced with a string literal during transformation if (platform_id == "cst") { if (param1 > 5) { out = 5; } else { if (in - param1 > 0){ out = in; } else { out = 10; } } } else { out = 0; }
This platform_id
parameter will be resolved in the
pre-compile
handler, being replaced with a string literal, and
as such will end up in the generated code.
What if a component is not supported by the platform?
// Generated from the component: Subsystem1.Gain1
// Component not supported for this platform. Outputs are zeroed.
_subsystem1_gain1__out = 0;
